Let’s look at how to access files stored in ArchiveLink and download them to the desktop. In the linked article, we discussed a way to write files to the SAP archive server. In this article, we will develop an ABAP program to read files from the archive server. First, we will look into the Function Modules (FM) needed to read files from ArchiveLink, then we will use those FMs to implement ABAP report that reads a given file from the archive content server and downloads it to the desktop.
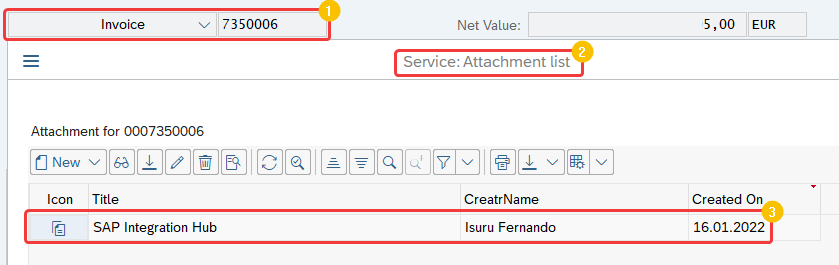
The screenshot above shows a PDF attachment that was archived by our custom ABAP program. In this article, we will learn how to programmatically retrieve the archived document from ArchiveLink.
Users can access the archived attachments using the Generic Object Service (GOS) toolbox of the business object.
The Design of the ABAP Program that Accesses ArchiveLink Files
This ABAP program accepts certain parameters related to the ArchiveLink document and downloads the file from the archive repository to the desktop. You can reuse the program to read any type of archived attachment from SAP business documents, such as purchase orders, invoices, sales orders, deliveries, etc.
If your requirement is to download the file to AL11, FTP, or convert the file content to base64, you can appropriate FMs instead of the GUI_DOWNLOAD. The rest of the ABAP processing steps to read attachments and files from ArchiveLink I have listed down will remain more or less the same.
Selection Screen
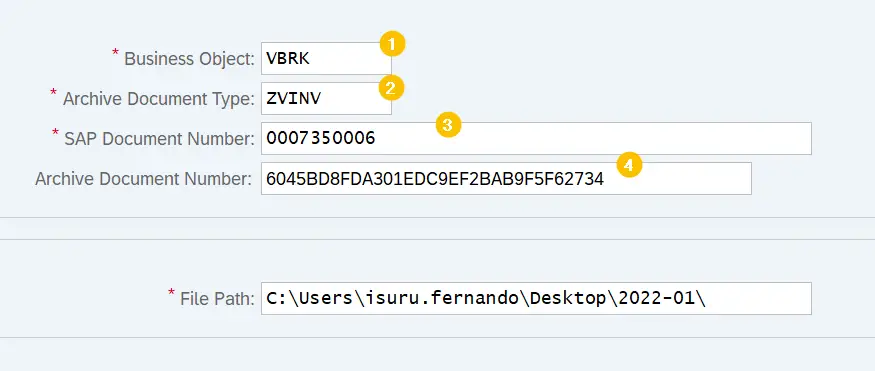
In our program, we have three main parameters related to ArchiveLink:
- SAP business object. Business object type of the SAP document, for example, VBRK for invoice, EKKO for purchase order, LIKP for delivery, VBAK for sales order, etc. To find a full list of business object types go to table TOJTB (Business object repository).
- ArchiveLink document type. For easier and logical segregation of files stored in the archive server, they are categorized into document types. Catagorization depends on your business requirment. This parameter correspons to field “AR_OBJECT” of table TOAOM (SAP ArchiveLink: Meta table for links).
- SAP document number. The document number of the business document which can be an invoice number, sales order number, purchase order number, etc. However, the document number should be a valid document number under the SAP business object of the first parameter.
- Archive document ID. ArchiveLink assigns a unique GUID for each and every document archived. Archive document ID is the unique identifier of each archived document. This parameter is related to field “ARC_DOC_ID” of link tables TOA01, TOA02 or TOA03.
Function Modules Used to Read from ArchiveLink
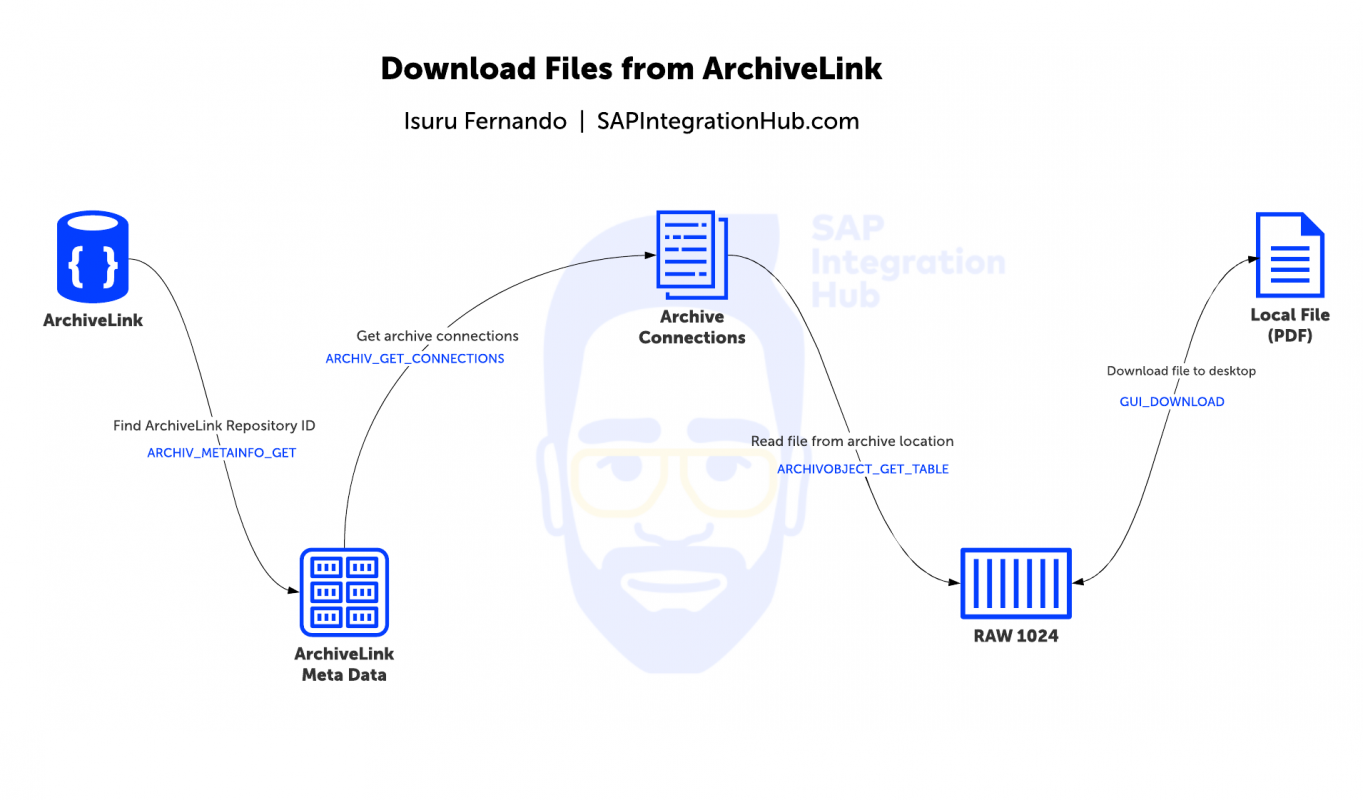
Step 1: As the first step, we will identify the ArchiveLink content repository ID based on the selection screen parameters, SAP business object, and archive document type. Pass the archive document type and sap business object type to FM ARCHIV_METAINFO_GET in order to find the archive server ID.
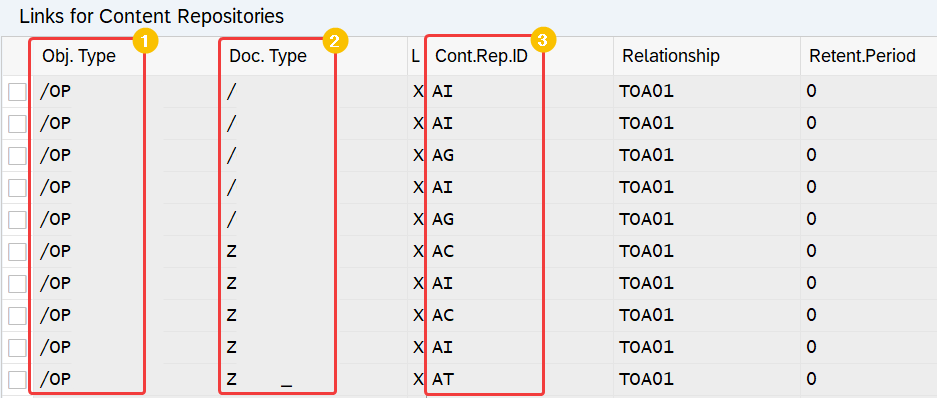
To view the full list of ArchiveLink repositories used for different SAP objects, go to transaction OAC3.
Step 2: The next step is to find out the list of file attachments archived under the SAP document. Use the FM ARCHIV_GET_CONNECTIONS to get a list of archived documents under the business document. The link between the business document and the archived document is known as ArchiveLink connection.
SAP link tables TOA01, TOA02, or TOA03 hold the full list of connections of each document.
In this example, we will read the first attachment from the list of archived attachments or use the archive document ID from the selection screen for further processing.
Step 3: Now that we have found out the archive document information from the previous step, let’s use the FM ARCHIVOBJECT_GET_TABLE to read the archived file from the content server. We will extract the file content in RAW 1024 format.
Step 4: Download the PDF to the desktop using GUI_DOWNLOAD.
ABAP Code of ArchiveLink Read Program
Main program
*&---------------------------------------------------------------------*
*& Report ZARCHIVE_FILE_READ
*&---------------------------------------------------------------------*
*& Author Isuru Fernando @ SAPIntegrationHub.com
*&---------------------------------------------------------------------*
REPORT zarchive_file_read.
DATA: gs_arc_meta TYPE toaom, "ArchiveLink meta data
gs_connections TYPE toav0. "Archived files linked to business document
DATA: gt_1024 TYPE TABLE OF tbl1024. "File in 1024 format
INCLUDE zarchive_file_read_forms.
SELECTION-SCREEN BEGIN OF BLOCK b1 WITH FRAME TITLE TEXT-002.
PARAMETERS: p_obj TYPE toav0-sap_object OBLIGATORY DEFAULT 'VBRK', "Business object type
p_obj_ty TYPE toaom-ar_object OBLIGATORY DEFAULT 'ZVINV', "Archive document type
p_doc_no TYPE toav0-object_id OBLIGATORY, "Business document number
p_arc_no TYPE toa01-arc_doc_id. "Archive document number
SELECTION-SCREEN END OF BLOCK b1.
SELECTION-SCREEN BEGIN OF BLOCK b2 WITH FRAME TITLE TEXT-001.
PARAMETERS: p_path TYPE string OBLIGATORY DEFAULT 'C:\Users\isuru.fernando\Desktop\2022-01\'. "File path
SELECTION-SCREEN END OF BLOCK b2.
END-OF-SELECTION.
PERFORM get_archive_repository USING p_obj_ty p_obj. "Find the archiveLink repository ID
PERFORM get_archive_connection_info USING p_obj p_doc_no CHANGING p_arc_no. "Find the archive files available for the given document
PERFORM read_file_from_archivelink USING p_obj_ty p_arc_no. "Read a file from archiveLink
PERFORM download_file USING p_path. "Download file to local PC
ABAP Include
*----------------------------------------------------------------------*
***INCLUDE ZARCHIVE_FILE_READ_FORMS.
*&---------------------------------------------------------------------*
*& Author Isuru Fernando @ SAPIntegrationHub.com
*&---------------------------------------------------------------------*
*----------------------------------------------------------------------*
*&---------------------------------------------------------------------*
*& Form GET_ARCHIVE_REPOSITORY
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> P_OBJ_TY
*& --> P_OBJ
*&---------------------------------------------------------------------*
FORM get_archive_repository USING p_obj_ty
p_obj.
DATA: lt_arc_meta TYPE TABLE OF toaom.
CALL FUNCTION 'ARCHIV_METAINFO_GET'
EXPORTING
* ACTIVE_FLAG = ' '
ar_object = p_obj_ty
sap_object = p_obj
TABLES
toaom_fkt = lt_arc_meta
EXCEPTIONS
error_connectiontable = 1
error_parameter = 2
OTHERS = 3.
IF sy-subrc <> 0.
PERFORM exception.
ELSE.
READ TABLE lt_arc_meta INTO gs_arc_meta INDEX 1.
ENDIF.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form GET_ARCHIVE_CONNECTION_INFO
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> P_OBJ
*& --> P_DOC_NO
*& --> P_ARC_DOC
*&---------------------------------------------------------------------*
FORM get_archive_connection_info USING p_obj
p_doc_no
CHANGING p_arc_no.
DATA: lt_toav0 TYPE TABLE OF toav0.
IF p_arc_no IS INITIAL.
CALL FUNCTION 'ARCHIV_GET_CONNECTIONS'
EXPORTING
objecttype = p_obj
object_id = p_doc_no
client = sy-mandt
archiv_id = gs_arc_meta-archiv_id
* ARC_DOC_ID =
* DOCUMENTTYPE =
* FROM_AR_DATE =
until_ar_date = sy-datum
* DOCUMENTCLASS =
* DEL_DATE =
* LIMITED =
* LIMIT =
* IMPORTING
* COUNT =
* REDUCEDBYLIMIT =
* REDUCEDBYAUTHORITY =
TABLES
connections = lt_toav0
* PARAMETER =
* FILE_ATTRIBUTES =
EXCEPTIONS
nothing_found = 1
OTHERS = 2.
IF sy-subrc <> 0.
PERFORM exception.
ELSE.
READ TABLE lt_toav0 INTO gs_connections INDEX 1.
p_arc_no = gs_connections-arc_doc_id.
ENDIF.
ENDIF.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form READ_FILE_FROM_ARCHIVELINK
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> P_OBJ_TY
*&---------------------------------------------------------------------*
FORM read_file_from_archivelink USING p_obj_ty p_arc_no.
DATA: lv_doc_type TYPE toadd-doc_type.
lv_doc_type = p_obj_ty.
CALL FUNCTION 'ARCHIVOBJECT_GET_TABLE'
EXPORTING
archiv_id = gs_arc_meta-archiv_id
document_type = lv_doc_type
archiv_doc_id = p_arc_no
** ALL_COMPONENTS =
signature = 'X'
** COMPID = 'data'
** IMPORTING
** LENGTH =
** BINLENGTH =
TABLES
** ARCHIVOBJECT =
binarchivobject = gt_1024
EXCEPTIONS
error_archiv = 1
error_communicationtable = 2
error_kernel = 3
OTHERS = 4.
IF sy-subrc <> 0.
PERFORM exception.
ENDIF.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form DOWNLOAD_FILE
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> P_PATH
*&---------------------------------------------------------------------*
FORM download_file USING p_path.
CONCATENATE p_path 'archive.pdf' INTO p_path.
CALL FUNCTION 'GUI_DOWNLOAD'
EXPORTING
* BIN_FILESIZE =
filename = p_path
filetype = 'BIN'
* APPEND = ' '
* WRITE_FIELD_SEPARATOR = ' '
* HEADER = '00'
* TRUNC_TRAILING_BLANKS = ' '
* WRITE_LF = 'X'
* COL_SELECT = ' '
* COL_SELECT_MASK = ' '
* DAT_MODE = ' '
* CONFIRM_OVERWRITE = ' '
* NO_AUTH_CHECK = ' '
* CODEPAGE = ' '
* IGNORE_CERR = ABAP_TRUE
* REPLACEMENT = '#'
* WRITE_BOM = ' '
* TRUNC_TRAILING_BLANKS_EOL = 'X'
* WK1_N_FORMAT = ' '
* WK1_N_SIZE = ' '
* WK1_T_FORMAT = ' '
* WK1_T_SIZE = ' '
* WRITE_LF_AFTER_LAST_LINE = ABAP_TRUE
* SHOW_TRANSFER_STATUS = ABAP_TRUE
* VIRUS_SCAN_PROFILE = '/SCET/GUI_DOWNLOAD'
* IMPORTING
* FILELENGTH =
TABLES
data_tab = gt_1024
* FIELDNAMES =
EXCEPTIONS
file_write_error = 1
no_batch = 2
gui_refuse_filetransfer = 3
invalid_type = 4
no_authority = 5
unknown_error = 6
header_not_allowed = 7
separator_not_allowed = 8
filesize_not_allowed = 9
header_too_long = 10
dp_error_create = 11
dp_error_send = 12
dp_error_write = 13
unknown_dp_error = 14
access_denied = 15
dp_out_of_memory = 16
disk_full = 17
dp_timeout = 18
file_not_found = 19
dataprovider_exception = 20
control_flush_error = 21
OTHERS = 22.
PERFORM exception.
ENDFORM.
FORM exception .
IF sy-subrc <> 0.
MESSAGE ID sy-msgid
TYPE sy-msgty
NUMBER sy-msgno
WITH sy-msgv1 sy-msgv2 sy-msgv3 sy-msgv4.
ENDIF.
ENDFORM.
To summarize, we’ve looked at the design of the ABAP program that reads files such as PDFs, TXT files, and images from ArchiveLink. Then, we’ve analyzed the FMs needed to read content from the archive repository. Finally, we have coded the ABAP program.
If you have questions or comments regarding ArchiveLink, please leave a message below.
SIGN UP TODAY!
Sign up to receive our monthly newsletter and special deals!