In this article, we will discuss the functionality of ArchiveLink, a data storage interface provided by SAP for archiving documents. We will first learn what the purpose of ArchiveLink is, then look into an ABAP program that can store files, such as PDFs, TXT, JPEG, in the archive content server. Additionally, we will study Function Modules (FM) for archiving documents using the ArchiveLink functionality.
If you like to learn how to read files from ArchiveLink, you can refer to the linked article.
What is ArchiveLink?
ArchiveLink is an interface provided by SAP that links SAP business documents and their attachments stored in a digital storage system. ArchiveLink allows us to store important documents, such as customer invoices, customs declarations, purchase orders, etc. in an internal or external data storage system. It also allows users to view the archived documents via SAP GUI transactions of business documents. For example, archived PDF attachments of sales orders can be accessed through the Generic Object Service (GOS) toolbox of transactions, sales order change (va02), sales order display(va03), etc.
ArchiveLink can store
- Incoming files – Files that are interfaced from external systems: scanned documents, emails, and files uploaded from local PCs.
- Outgoing files – Files created in SAP system, such as outputs of SAPScripts and Smartforms.
- Print Lists – Results of report outputs
- Archive Files
ABAP Program – Write Files to ArchiveLink
Let’s design an ABAP program that can upload files from a local PC and store them in an archive server using ArchiveLink. We will use selection parameters to link the file uploaded to an SAP business document such as invoice, sales order, purchase order, etc.
Selection Screen of the ABAP Program
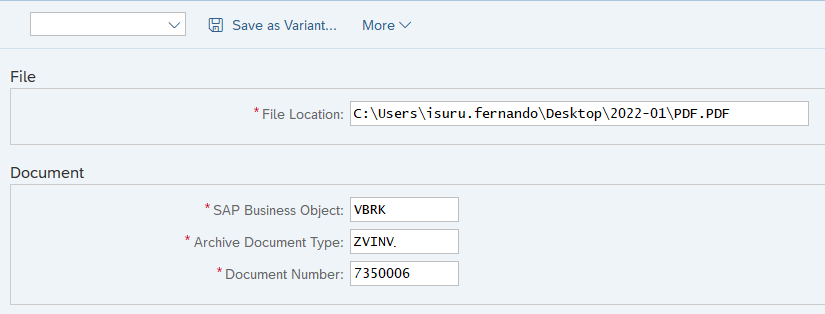
Parameters of the selection screen are as follows,
- File location – Full path of the file to be uploaded.
- SAP business object – Corresponds to the SAP business object type. For example, VBRK for billing document, VBAK for sales orders, etc.
- Archive document type – The document type of the uploaded file. This parameter corresponds to the archive document type configured under transation OAC2.
- Document number – The document number of the SAP business object.
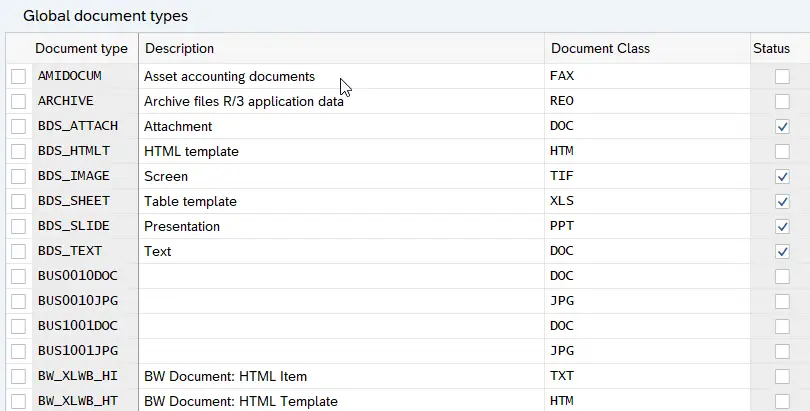
Output of the ArchiveLink Processing Program
The primary purpose of the program is to store the uploaded file into the archive repository. Additionally, it should display the stored archive file as an attachment in the GOS toolbox of the transactions. In this example, the PDF we store in ArchiveLink should be visible in the attachment list of the invoice.
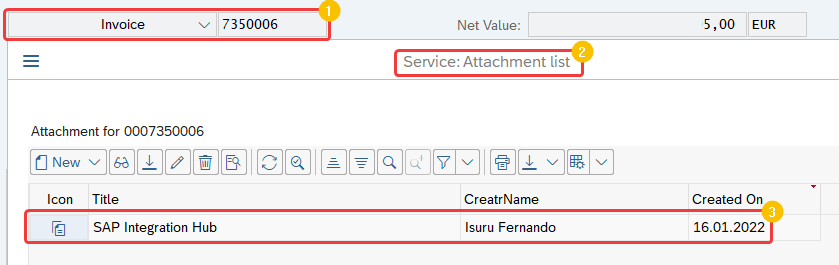
ArchiveLink File Processing Logic and Function Modules
Now we will design the ABAP program that writes files in ArchiveLink. We will look into the data processing steps and the function modules used for ArchiveLink.
- The first step of the processing logic is to upload the file from local PC. We will use GUI_UPLOAD to upload the file content into SAP Office binary format.
- Next, convert the binary format of the file content to Xstring via function module SCMS_BINARY_TO_XSTRING.
- Then, convert the Xstring to RAW 1024 format. RAW 1024 is the data format accepted by ArchiveLink FM which writes the data to data storage. Use the FM SCMS_XSTRING_TO_BINARY to convert the data to RAW 1024 binary format.
- Aftwards, use SAP business object type and archive document type to find the archive content repository. For this, you can use the FM ARCHIV_CONNECTDEFINITION_GET.
- Next step is to define a new archive document ID for the file that we will store. You can use the FM ARCHIVOBJECT_CREATE_TABLE for this purpose.
- Finally, link the business document (invoice) with the archive document ID using function module ARCHIV_CONNECTION_INSERT.
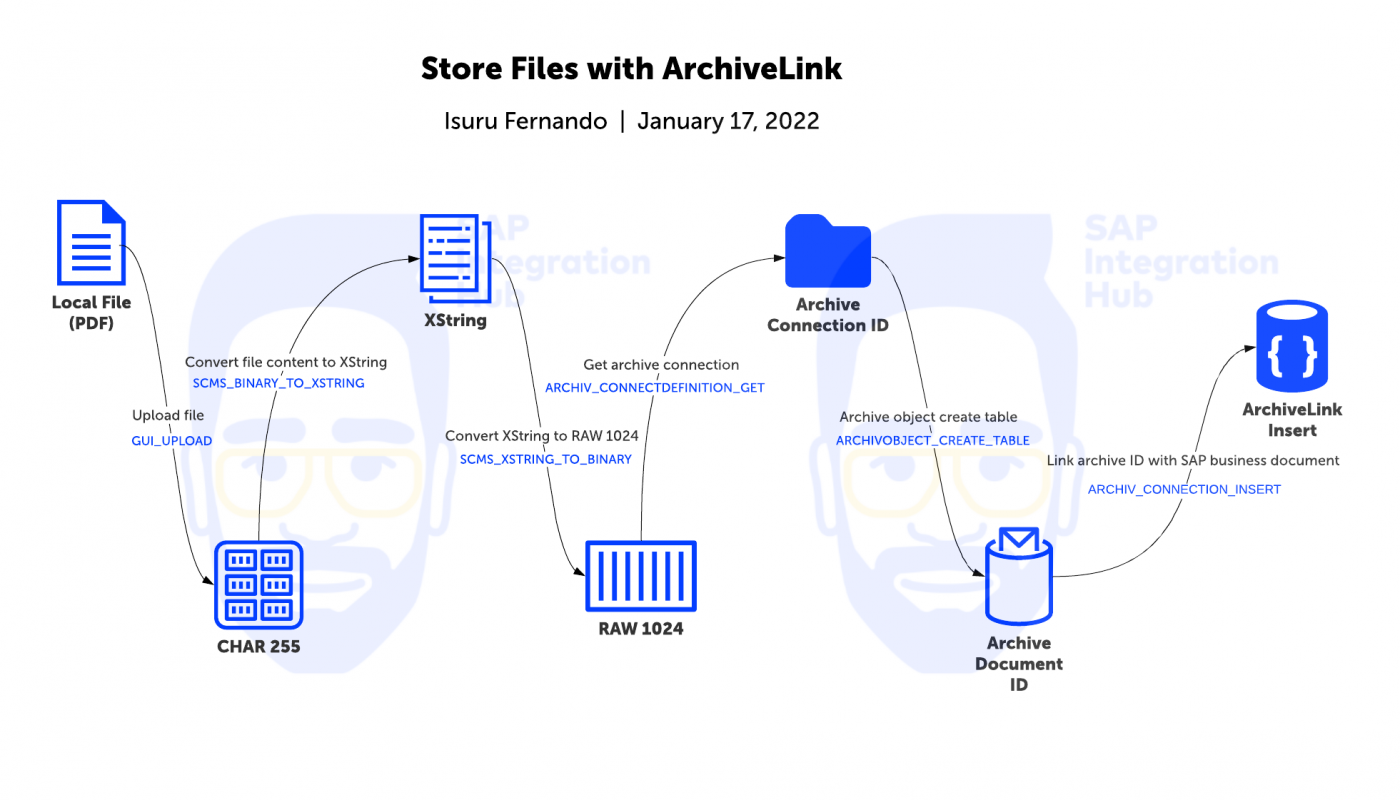
Refer to the linked article if your requirement involves archiving files transmitted in base64 format.
ABAP Program Code
Main Program
*&---------------------------------------------------------------------*
*& Report ZARCHIVE_FILE
*&---------------------------------------------------------------------*
*& Author Isuru Fernando @ SAPIntegrationHub.com
*&---------------------------------------------------------------------*
REPORT zarchive_file.
DATA: gt_file_table TYPE filetable, "Uploaded file information
gt_content TYPE soli_tab, "Uploaded files content
gv_len TYPE i. "File length
DATA: gv_ext TYPE sood1-file_ext, "File extension
gv_fname TYPE sood1-objdes. "File name
data: gv_archiv_id TYPE saearchivi, "Archive connection ID
gv_xstring TYPE xstring, "File in Xstring
gv_out TYPE i,
gv_arc_doc_id TYPE saeardoid. "Archive document ID
DATA gt_content1024 TYPE TABLE OF tbl1024. "RAW 1024 format
INCLUDE zarchive_file_forms.
SELECTION-SCREEN BEGIN OF BLOCK b1 WITH FRAME TITLE TEXT-001.
PARAMETERS: p_path TYPE string OBLIGATORY. "File path
SELECTION-SCREEN END OF BLOCK b1.
SELECTION-SCREEN BEGIN OF BLOCK b2 WITH FRAME TITLE TEXT-002.
PARAMETERS: p_obj TYPE toav0-sap_object OBLIGATORY DEFAULT 'VBRK', "Business object type
p_obj_ty TYPE toaom-ar_object OBLIGATORY DEFAULT 'ZVINV', "Archive document type
p_doc_no TYPE vbeln OBLIGATORY. "Business document number
SELECTION-SCREEN END OF BLOCK b2.
AT SELECTION-SCREEN ON VALUE-REQUEST FOR p_path.
PERFORM open_file_dialog USING p_path. "Open file upload dialog box
START-OF-SELECTION.
PERFORM upload_file USING p_path. "Upload file
PERFORM split_file_path USING p_path. "Find file extension and file name
PERFORM get_archive_connection USING p_obj p_obj_ty. "Get carchive connection of SAP object and archive document type
PERFORM convert_binary_to_xstring. "Convert binary to Xstring
PERFORM convert_xstring_to_1024. "Convert Xstring to raw 1024
PERFORM archive_obj_create_table. "Create archive table link and create archive document ID
PERFORM archive_obj_insert USING p_obj p_obj_ty p_doc_no. "Save archive document
Include
*&---------------------------------------------------------------------*
*& Include ZATTACH_FILE_FORMS
*&---------------------------------------------------------------------*
*&---------------------------------------------------------------------*
*& Form OPEN_FILE_DIALOG
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> p1 text
*& <-- p2 text
*&---------------------------------------------------------------------*
FORM open_file_dialog USING p_path.
DATA: ls_file_table LIKE LINE OF gt_file_table,
lv_rc TYPE i.
CLEAR p_path.
REFRESH: gt_file_table.
CALL METHOD cl_gui_frontend_services=>file_open_dialog
EXPORTING
window_title = 'Select File'
default_filename = '*.pdf'
multiselection = 'X'
CHANGING
file_table = gt_file_table
rc = lv_rc.
READ TABLE gt_file_table INDEX 1 INTO ls_file_table.
p_path = ls_file_table-filename.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form UPLOAD_FILE
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> p1 text
*& <-- p2 text
*&---------------------------------------------------------------------*
FORM upload_file USING p_path.
CALL FUNCTION 'GUI_UPLOAD'
EXPORTING
filename = p_path
filetype = 'BIN'
IMPORTING
filelength = gv_len
TABLES
data_tab = gt_content.
PERFORM exception.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form SPLIT_FILE_PATH
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> P_PATH
*& --> P_EXT
*& --> P_FNAME
*&---------------------------------------------------------------------*
FORM split_file_path USING p_path.
DATA: lv_filename TYPE pcfile-path.
CHECK p_path IS NOT INITIAL.
lv_filename = p_path.
CALL FUNCTION 'PC_SPLIT_COMPLETE_FILENAME'
EXPORTING
complete_filename = lv_filename
* CHECK_DOS_FORMAT =
IMPORTING
* DRIVE =
extension = gv_ext
* NAME =
name_with_ext = gv_fname
* PATH =
EXCEPTIONS
invalid_drive = 1
invalid_extension = 2
invalid_name = 3
invalid_path = 4
OTHERS = 5.
PERFORM exception.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form EXCEPTION
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> p1 text
*& <-- p2 text
*&---------------------------------------------------------------------*
FORM exception .
IF sy-subrc <> 0.
MESSAGE ID sy-msgid
TYPE sy-msgty
NUMBER sy-msgno
WITH sy-msgv1 sy-msgv2 sy-msgv3 sy-msgv4.
ENDIF.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form GET_ARCHIVE_CONNECTION
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> P_OBJ
*& --> P_OBJ_TY
*&---------------------------------------------------------------------*
FORM get_archive_connection USING p_obj
p_obj_ty.
CALL FUNCTION 'ARCHIV_CONNECTDEFINITION_GET'
EXPORTING
objecttype = p_obj
documenttype = p_obj_ty
client = sy-mandt
IMPORTING
archivid = gv_archiv_id
EXCEPTIONS
nothing_found = 1
OTHERS = 2.
PERFORM exception.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form CONVERT_BINARY_TO_XSTRING
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> p1 text
*& <-- p2 text
*&---------------------------------------------------------------------*
FORM convert_binary_to_xstring .
CALL FUNCTION 'SCMS_BINARY_TO_XSTRING'
EXPORTING
input_length = gv_len
* FIRST_LINE = 0
* LAST_LINE = 0
IMPORTING
buffer = gv_xstring
TABLES
binary_tab = gt_content.
PERFORM exception.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form CONVERT_XSTRING_TO_1024
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> p1 text
*& <-- p2 text
*&---------------------------------------------------------------------*
FORM convert_xstring_to_1024 .
CALL FUNCTION 'SCMS_XSTRING_TO_BINARY'
EXPORTING
buffer = gv_xstring
IMPORTING
output_length = gv_out
TABLES
binary_tab = gt_content1024[].
PERFORM exception.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form ARCHIVE_OBJ_CREATE_TABLE
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> p1 text
*& <-- p2 text
*&---------------------------------------------------------------------*
FORM archive_obj_create_table .
DATA: lv_doc_typ TYPE toadv-doc_type,
lv_arc_length TYPE sapb-length.
lv_doc_typ = gv_ext. "File extension
lv_arc_length = gv_len. "File length
CALL FUNCTION 'ARCHIVOBJECT_CREATE_TABLE'
EXPORTING
archiv_id = gv_archiv_id
document_type = lv_doc_typ
length = lv_arc_length
* vscan_profile = lv_vscan_profile
*** compid = 'data'
IMPORTING
archiv_doc_id = gv_arc_doc_id
TABLES
binarchivobject = gt_content1024
* archivobject = lt_content2
EXCEPTIONS
error_archiv = 1
error_communicationtable = 2
error_kernel = 3
OTHERS = 4.
PERFORM exception.
ENDFORM.
*&---------------------------------------------------------------------*
*& Form ARCHIVE_OBJ_INSERT
*&---------------------------------------------------------------------*
*& text
*&---------------------------------------------------------------------*
*& --> p1 text
*& <-- p2 text
*&---------------------------------------------------------------------*
FORM archive_obj_insert USING p_obj p_obj_ty p_doc_no.
DATA: lv_doc_type TYPE toadv-doc_type,
lv_file_name TYPE toaat-filename,
lv_object_no TYPE sapb-sapobjid.
lv_doc_type = gv_ext. "File type PDF
lv_file_name = gv_fname. "File Name
lv_object_no = p_doc_no. "Invoice number
CALL FUNCTION 'ARCHIV_CONNECTION_INSERT'
EXPORTING
archiv_id = gv_archiv_id
arc_doc_id = gv_arc_doc_id
ar_object = p_obj_ty
object_id = lv_object_no
sap_object = p_obj
doc_type = lv_doc_type
filename = lv_file_name
descr = 'SAP Integration Hub'
creator = sy-uname
EXCEPTIONS
error_connectiontable = 1
OTHERS = 2.
PERFORM exception.
ENDFORM.
To summarize, we talked about the purpose of ArchiveLink, then looked into how to modularize an ABAP program to store documents, such as PDFs, TXT, images, in the archive repository. Additionally, we developed a custom ABAP program that can write files to the ArchiveLink repository and link the archive files to SAP business documents.
If you have any comments or suggestions on the topic, please post below.
Hi,
I have tried your solution to archive vendor invoices, it successfully uploaded the document in archivelink but unfortunately, I am not able to retrieve the document in FB03, the PDF File is corrupted.
Do you have any suggestions to rectify this issue so that I can see the pdf whenever needed ?