What is a Fault Message? Fault Messages provide information about application-specific errors that occur in the inbound system. Think about Fault Messages as an acknowledgment from the message receiver to the message sender or the message monitor about the application processing status.
Application-specific errors are errors that occur in the application processing based on business rules. In this article, we will discuss use cases, structure, and implementation steps in detail and learn how to monitor Fault Messages.
SAP Versions used in the illustration:
- SAP S4 HANA Fashion 1709
- SAP PO 7.5
Use Cases of Fault Messages in ABAP Proxy
Use case 1: Capturing BAPI/FM Return
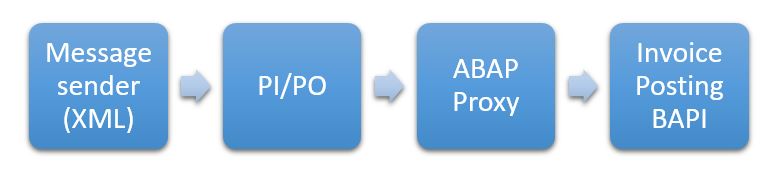
Let’s assume a sender system sends a message with invoice information to target system SAP. The invoice is posted from the inbound ABAP proxy class using a BAPI.
What will happen if all information needed to post an invoice in SAP is not sent in the message? Obviously, invoice won’t be posted in the target SAP system. But how does a user identify the issue? What are the return messages from BAPI? If we do not capture the BAPI return messages and pass it back to the message monitor, messages will always show as successful in SXMB_MONI as technically message was processed successfully. (!!!message message messaged success successful)
Keep in mind we are not talking about missing mandatory values in the message. If all mandatory fields filled in the sender message are not populated, either the XML validation or message mapping will throw out a technical error.
We are talking about how to capture application processing issues (BAPI return messages) and send the status of the invoice processing to message monitor. Then, the user will be able to identify application processing (Invoice posting) errors and take necessary actions to correct the issue and reprocess the messages.
To give you another example, let’s assume the FI posting period is not open in SAP. The invoice will not be posted. If fault messages are not captured from the BAPI return, SXMB_MONI will show the message as successfully processed.
Use Case 2: Message Validations
Let’s assume you need to validate the inbound message content based on business rules before processing the message in SAP. How do we capture the status of the validation in message monitor?
If validation failures are not passed to SXMB_MONI as an exception, the message will always show as successfully processed. In this case, business users won’t be able to identify which messages actually posted successfully in SAP and which messages did not pass the validation.
Therefore, you need to capture error messages of the validation and raise them as an exception.
For example, take the exchange rates interface we built previously. This interface updates exchange rates in SAP via a BAPI implemented in the proxy class. Now we have a requirement to validate the message content before calling the BAPI. “Valid from date” in the exchange rates input message should not be in the past.
Structure or Format of Fault Messages in SAP PI and ABAP Proxy
Standard Fault Messages in SAP PI/PO have a predefined format. But of course you can enhance this format to add additional fields and segments if necessary.
Standard Structure of Fault Messages in SAP PI
Standard Fault Message Type has 3 main segments. They are two fields named faultText and faultUrl and a table named faultDetail.
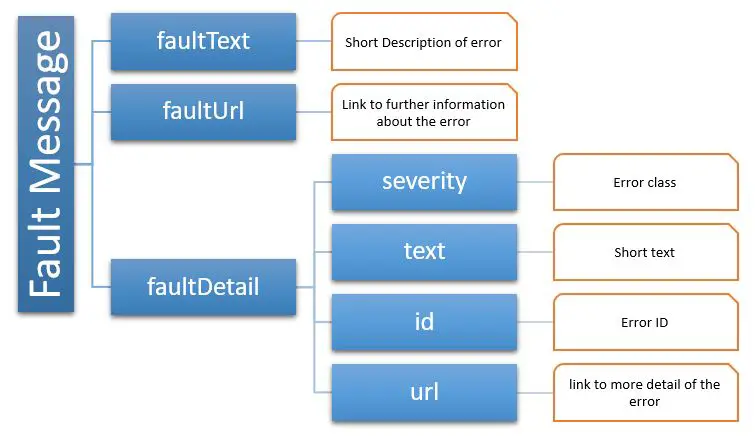
faultText:
This parameter provides a short description of the application error. It provides an overall status of message processing. For example, for use case 1, we can define messages as “Invoice not posted”.
In use case 2, we can define them as “Exchange rates not updated in SAP”
faultUrl:
This is the Url where user can find more information about the error in faultText.
faultDetail:
This is a table parameter which can hold multiple error/warning/information messages from the application processing. For example, BAPI can return multiple messages about invoice processing or application processing. We can include all those messages to the proxy run-time monitor so the user can find all the details of the message processing.
Structure of the faultDetail includes four fields:
- severity
- text
- url
- id
severity
You can define the severity of the message in this field. Message types such as E (Error), I (information), W (Warning), S (Success) can be assigned to this field. This correlates to the messages type in ABAP MSGTY.
text
Message text.
url
Url to the long text of the message in ‘text‘.
id
You can assign the message ID here. For example, message class and message number of the error in SAP. Assuming our message class is SD and message ID is 002, you can set the ID as SD(002).
This correlates to how the message class and message numbers are defined in transaction se91.
Example of Fault Message Implementation
To demonstrate the functionality of Fault Messages I will re-use the exchange rates interface file to proxy example. This interface updates the exchange rates in SAP using function module ‘BAPI_EXCHANGERATE_CREATE’.
Have a look at the implementation of the exchange rates interface before proceeding.
We will modify the exchange rates interface to return application processing errors to SXMB_MONI. Let’s assume if the “Valid from” date (VALID_FROM) of the exchange rates record in the file is in the past, we do not want to update exchange rates in SAP. Records which do not satisfy the “Valid from” date validation should be visible in SXMB_MONI as error messages.
Pseudo Code of the Validation Logic in Proxy Class
“Valid from date” validation should be implemented in the Proxy class. Here’s how the processing logic looks like.
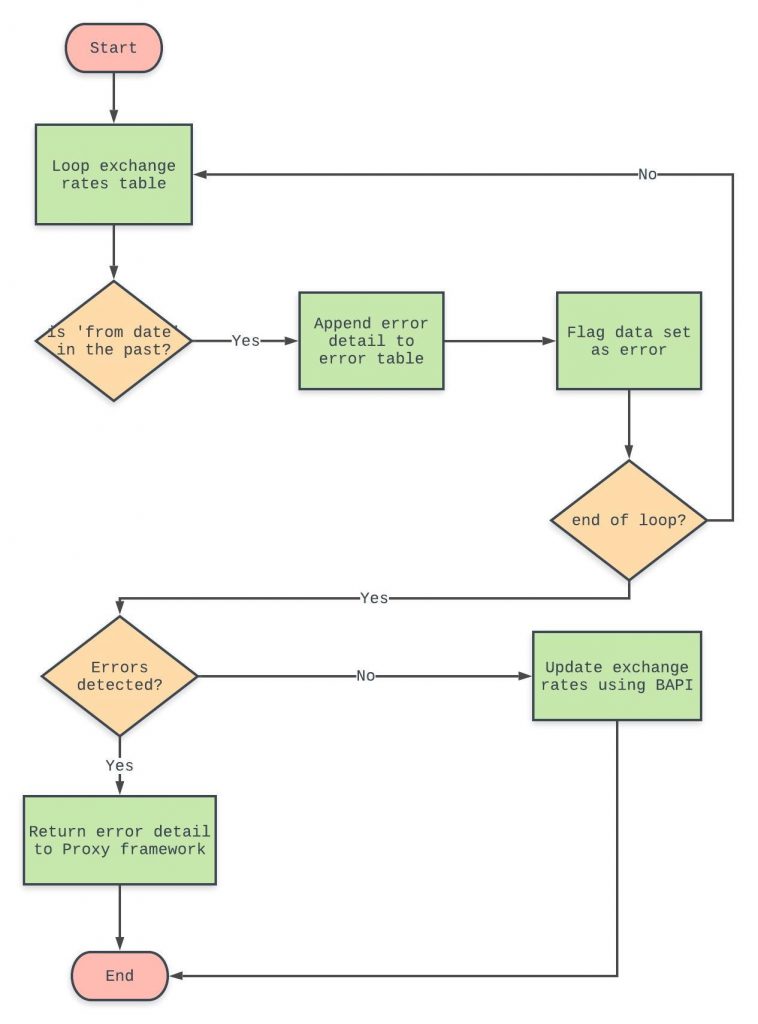
Fault Messages Implementation Steps
Now we will look at all the steps of Fault Messages implementation in an inbound interface. The differences in steps between the Asynchronous Proxy interface we previously implemented without Fault Message and this interface with Fault Messages are,
- Creation of Data Type and a Message Type of standard Fault Message type.
- Changing the Inbound Service Interface to reflect response Fault Data.
- Creation of a new Operation Mapping.
Full list of Fault Message implementation steps are as follows,
- Configure Proxy connectivity between SAP PI/PO and SAP back-end system.
- Create standard Fault Message’s Data Type and Message Type.
- Create sender Data Type and Message Type.
- Create receiver Proxy Data Type and Message Type.
- Define the Inbound Service Interface with Fault Message.
- Implement Message Mapping and Operation Mapping.
- Generate Proxy Class in SAP back-end system.
- Implement ABAP logic to return application errors to SXMB_MONI.
- Configure the iFlow.
As all the steps, except for step 2, step 5, and step 8, are the same for Fault Message implementation as the original exchange rates interface, I will demonstrate only the detail of steps 2, 5 and 8 in this article. You can refer to the previous post to check more information about other steps.
Step 2: Create Fault Message
First, create two data types for FaultData and Log Data. You can copy any standard fault message data types to your custom SWCV and namespace.
Data Type ExchangeLogData
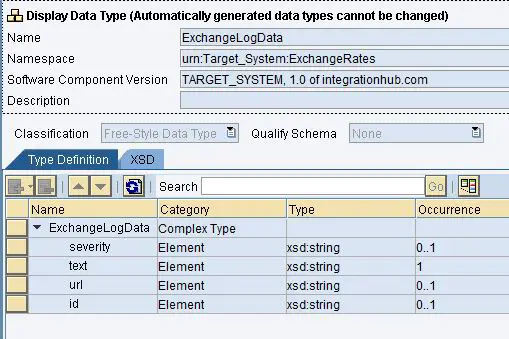
Data Type ExchangeFaultData
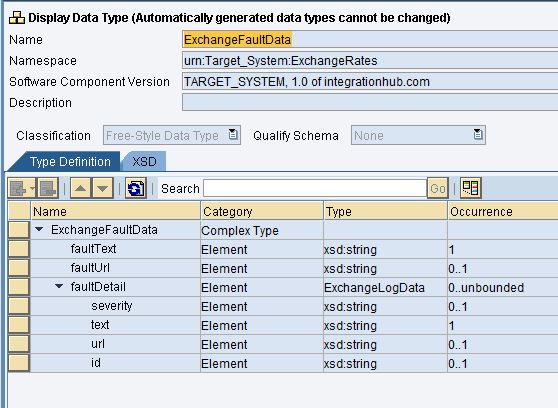
Create Fault Message Type
Create a Fault Message Type by assigning the Fault Data Type ExchangeFaultData created previously.
To create the Fault Message Type, first, right-click on the namespace and select “Fault Message Type” from the list of objects.
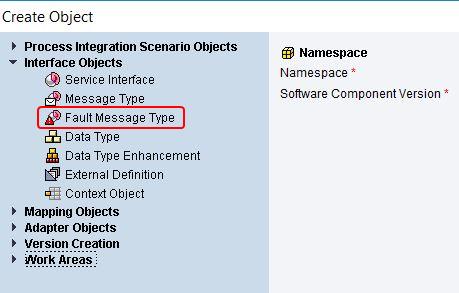
Then assign the Data Type ExchangeFaultData we created in step 2 to the Fault Message Type.
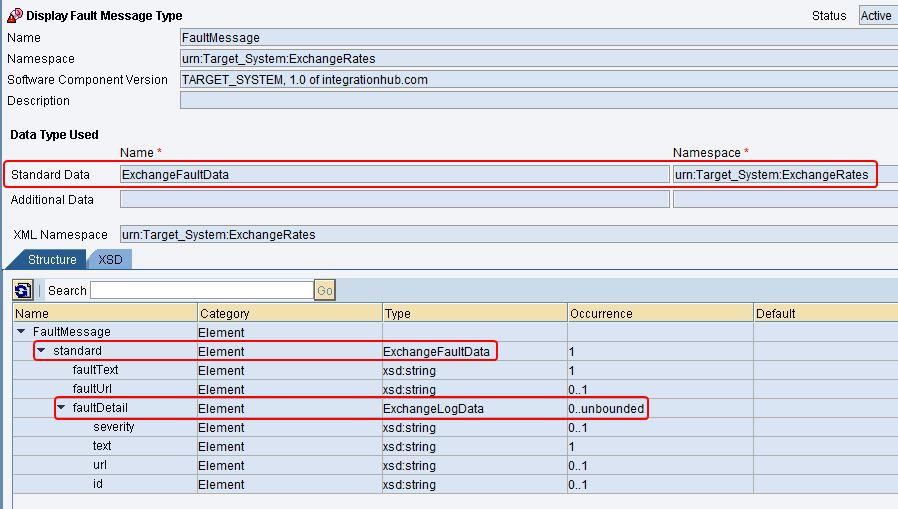
Step 5: Define the Inbound Service Interface with Fault Message
Create the Inbound Service Interface and assign the Fault Message Type we created in Step 3.
The name of the Inbound Service Interface in this example is ‘ExchangeRateswithFaultMessage_Inb_Async’.
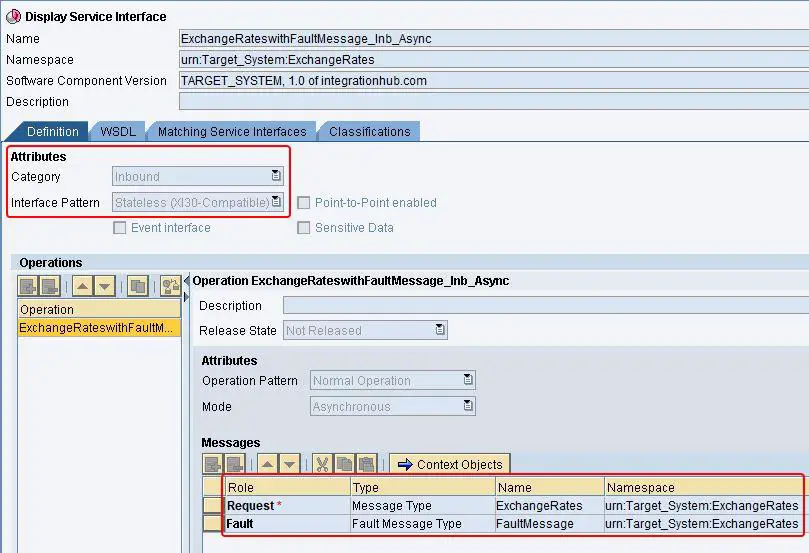
Step 8: Implement ABAP logic in Proxy Class
Create the Inbound proxy class the usual way using SPROXY transaction.
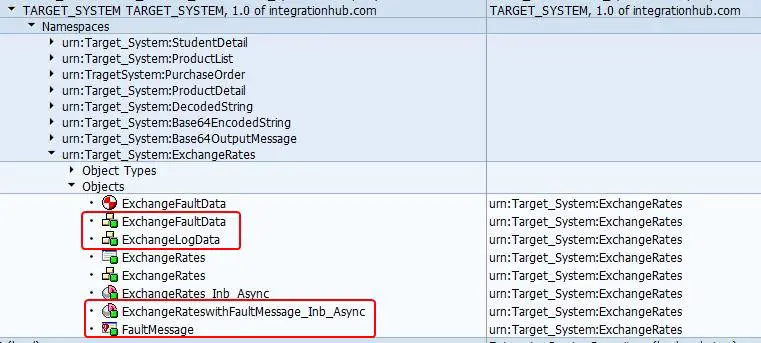
Go to Proxy implementation class and you will be able to view the exception class ZCX_FAULT_MESSAGE in the method signature.
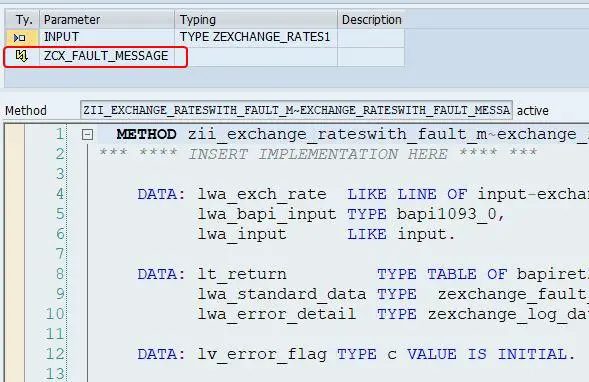
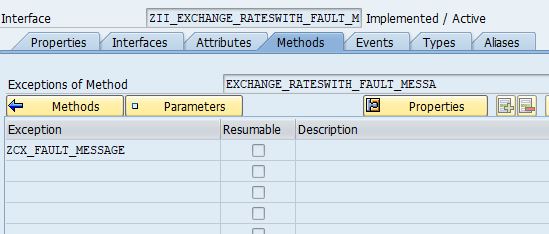
Notice that the Fault Message Type we created in SAP PI/PO ESR is generated as a DDIC structure in SAP back end.
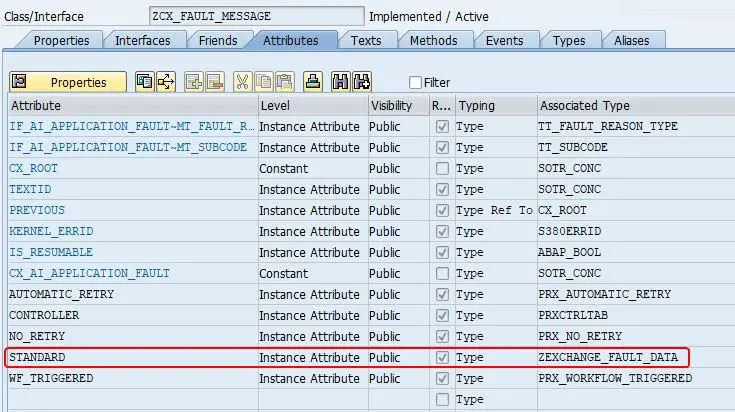
DDIC structure ZEXCHANGE_FAULT_DATA correlates to FaultMessage Message Type in ESR.
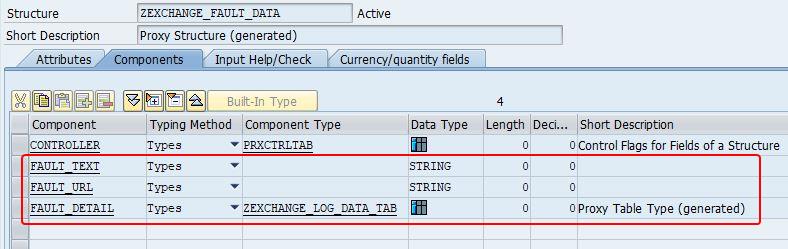
Also, DDIC structure ZEXCHANGE_LOG_DATA corresponds to ExchangeLogData Data Type.
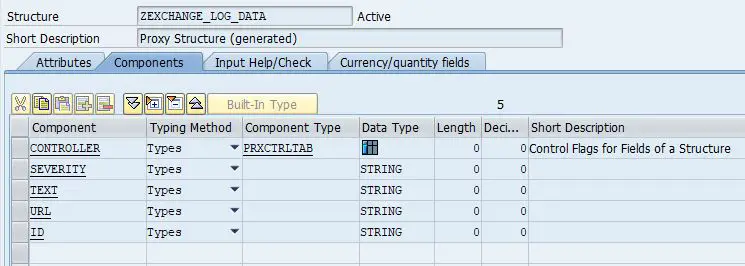
Proxy Class ABAP Code
METHOD zii_exchange_rateswith_fault_m~exchange_rateswith_fault_messa. *** **** INSERT IMPLEMENTATION HERE **** *** DATA: lwa_exch_rate LIKE LINE OF input-exchange_rates-exch_rate, lwa_bapi_input TYPE bapi1093_0, lwa_input LIKE input. DATA: lt_return TYPE TABLE OF bapiret2, lwa_standard_data TYPE zexchange_fault_data, lwa_error_detail TYPE zexchange_log_data. DATA: lv_error_flag TYPE c VALUE IS INITIAL. *Assign proxy input message to a local variable lwa_input = input. *Validate if data is in the past *Loop data records of in coming proxy message LOOP AT lwa_input-exchange_rates-exch_rate INTO lwa_exch_rate. *Check if from date is in the past IF lwa_exch_rate-valid_from < sy-datum. lv_error_flag = abap_true. *Append errors to exception table lwa_standard_data-fault_text = 'Error Ooccured, exchanges rates were not posted in SAP'. lwa_standard_data-fault_url = 'https://sapintegrationhub.com'. CLEAR lwa_error_detail. lwa_error_detail-severity = 'High'. CONCATENATE 'Date' lwa_exch_rate-valid_from 'is in the past' INTO lwa_error_detail-text SEPARATED BY space. lwa_error_detail-id = sy-tabix. "Record number of the input message lwa_error_detail-url = 'https://sapintegrationhub.com'. APPEND lwa_error_detail TO lwa_standard_data-fault_detail. ENDIF. ENDLOOP. *if no errors found update the exchanges rates if lv_error_flag is INITIAL. LOOP AT lwa_input-exchange_rates-exch_rate INTO lwa_exch_rate. MOVE-CORRESPONDING lwa_exch_rate TO lwa_bapi_input. CALL FUNCTION 'BAPI_EXCHANGERATE_CREATE' EXPORTING exch_rate = lwa_exch_rate upd_allow = 'X'. ENDLOOP. *Else send acknowledgment ELSEIF lv_error_flag = abap_true. RAISE EXCEPTION TYPE zcx_fault_message EXPORTING * textid = * * previous = * * automatic_retry = * * controller = * * no_retry = standard = lwa_standard_data. ENDIF. ENDMETHOD.
Test Fault Message using PI/PO Test Tool
Test Case
We will trigger a message with valid date in the past. In the test message <VALID_FROM> date is 2019-05-01 which is in the past.
Test Message
<?xml version="1.0" encoding="UTF-8"?> <ns1:ExchangeRates xmlns:ns1="urn:Target_System:ExchangeRates"> <EXCH_RATE> <RATE_TYPE>M</RATE_TYPE> <FROM_CURR>USD</FROM_CURR> <TO_CURRNCY>EUR</TO_CURRNCY> <VALID_FROM>2019-05-01</VALID_FROM> <EXCH_RATE>0.89</EXCH_RATE> <FROM_FACTOR>1</FROM_FACTOR> <TO_FACTOR>1</TO_FACTOR> <EXCH_RATE_V>0.0</EXCH_RATE_V> <FROM_FACTOR_V>0</FROM_FACTOR_V> <TO_FACTOR_V>0</TO_FACTOR_V> </EXCH_RATE> </ns1:ExchangeRates>
Monitor Interface Using SXMB_MONI
Go to transaction SXMB_MONI to monitor the application processing status of the message. You will notice it has been flagged as an error message.
You will be able to see the message in status “Application Error – Manual Restart Possible”.
If you need to analyze the behavior of the Proxy ABAP logic at runtime, set an external debugging break-point and trigger the interface from PI.
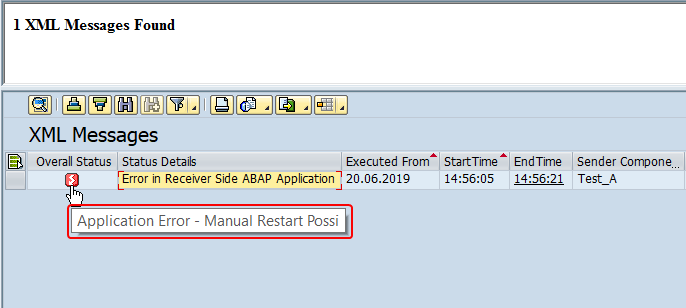
Business users will be able to easily identify messages which were not posted in SAP due to ABAP validation not being satisfied. While messages which processed successfully will be in a successful status while messages failed due to validation will be flagged in an error status.
How to Monitor Fault Message Details in SXMB_MONI?
To view the message processing detail and payload, double click on the message in SXMB_MONI to go to the detail view.
You can view the Inbound Message and Call Inbound Proxy messages in the navigation tree.
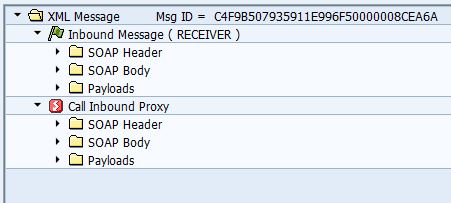
Go to Main Document section of Call Inbound Proxy message to view the error detail. This detail you can view here, we appended from the ABAP proxy logic.
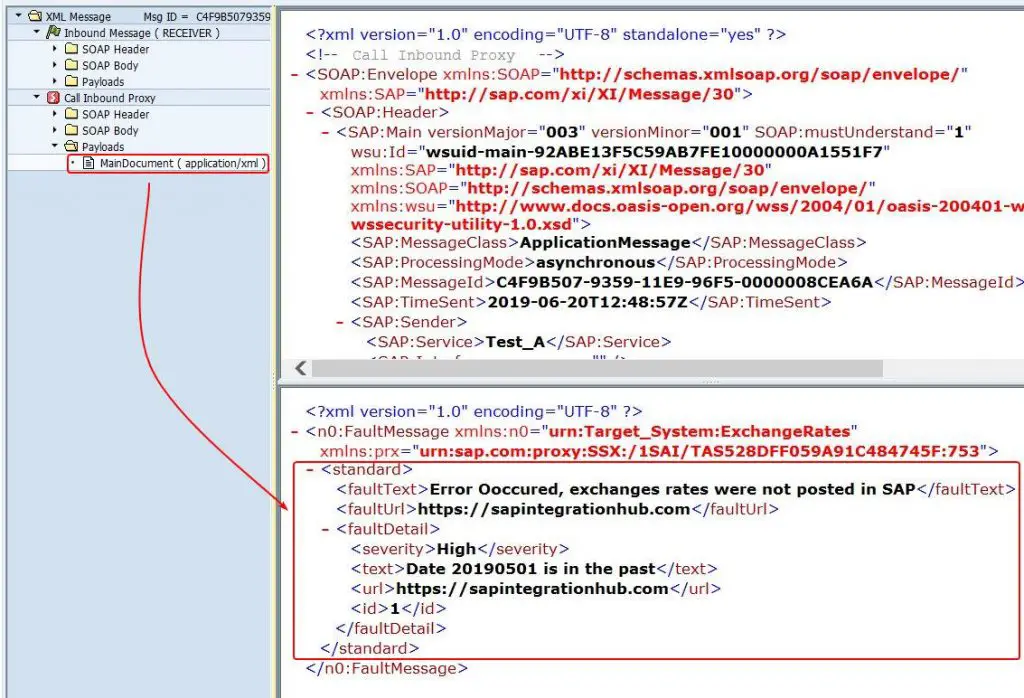
Hope this illustration cleared any doubts you had about the use of Fault Messages in SAP. If you have any questions, please leave a comment below!
Hi Isuru,
Good post! Currently stuck in a situation. The flow is 3rd Party(REST) -> PO -> ECC. My requirement is to return a code when ECC is down. I understand this is possible through fault messages, but I don’t see how. I have done the PI part, but how can the ABAP part of fault message be configured to return something when the whole ECC is down? Any advice is much appreciated 🙂
Hi Isuru,
Good post! Currently stuck in a situation. The flow is 3rd Party(REST) -> PO -> ECC. My requirement is to return a code when ECC is down. I understand this is possible through fault messages, but I don’t see how. I have done the PI part, but how can the ABAP part of fault message be configured to return something when the whole ECC is down? Any advice is much appreciated 🙂
Hi Isuru,
Great post!!
Any idea if I need the async fault message back to SAP PI.
Regards,
Hi Isuru,
Great post!!
Any idea if I need the async fault message back to SAP PI.
Regards,
Hello Gurpreet,
Try using the channel parameter ‘XMBWS.NoSOAPIgnoreStatusCode’. Check if this thread would help.
Cheers!
Isuru
Hi! Would you have an idea why there is a call failed? we followed the steps, but we’re having call failed message.
Hi Marie,
Could you send me a screenshot of the message?