This article provides a sample Java mapping code to decode Base64 string in SAP PI/PO. You can decode Base64 string in SAP PI/PO with User-Defined Function (UDF), Java Mapping and Adapter Modules. In this example, we will discuss how to achieve Base64 conversion using Java Mapping. Feel free to read my previous article on Base64 encoding and decoding methods in SAP ABAP. Additionally, you can refer to my SAP blog on the same subject.
Moreover, I have implemented an example interface with a Base64 encoding java mapping.
SAP Versions used in the illustration:
- SAP PO 7.5
Base64 Decoding Example Scenario:
Let’s assume, a sender message contains Base64 string and we need to assign the decoded string to the target message.
Source message xml node <Base64EncodedString> contains the encoded message. Decoded string should be mapped to node <Base64DecodedString> of the target message.
This example will be implemented in an SAP Process Integration (PI) system version 7.50 single stack.
Expected Functionality of Java Mapping:
Final result with input message and desired output message are as below.
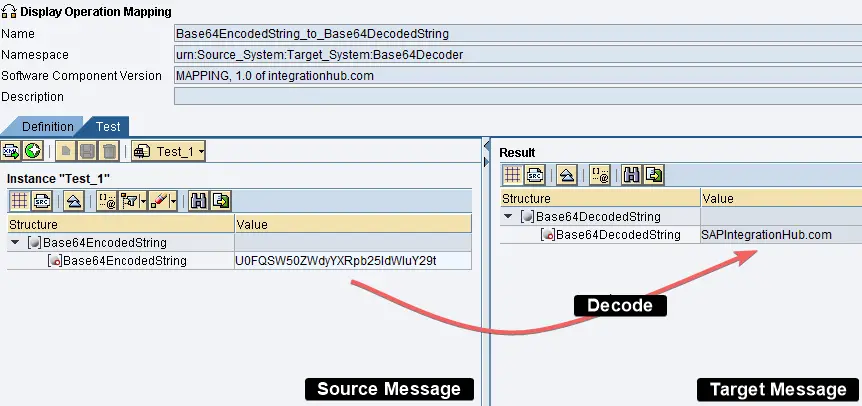
The first function of Java Mapping is to convert Base64 encoded string in ‘Base64EncodedString’.
Encoded string ‘U0FQSW50ZWdyYXRpb25IdWIuY29t’ translates to ‘SAPIntegrationHub.com’.
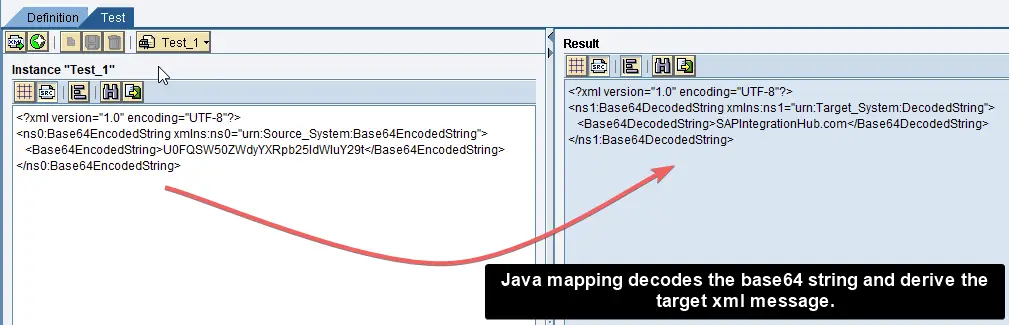
Next, Java Mapping should form the output or target xml message.
Input Message:
<?xml version="1.0" encoding="UTF-8"?> <ns0:Base64EncodedString xmlns:ns0="urn:Source_System:Base64EncodedString"> <Base64EncodedString>U0FQSW50ZWdyYXRpb25IdWIuY29t</Base64EncodedString> </ns0:Base64EncodedString>
Output Message:
<?xml version="1.0" encoding="UTF-8"?> <ns1:Base64DecodedString xmlns:ns1="urn:Target_System:DecodedString"> <Base64DecodedString>SAPIntegrationHub.com</Base64DecodedString> </ns1:Base64DecodedString>
Steps to Implement Base64 Message Mapping in Enterprise Service Repository:
- Create Data Types, Message Types, and Service Interfaces.
- Create Base64 Java Mapping in Netweaver Development Studio (NWDS).
- Implement Operation Mapping (OM).
Step 1: Create Data Types, Message Types and Service Interfaces.
Create these ESR objects to match the scenario. We have two Data types and two Message types each for sender and receiver.
Data types and Message types are defined to reflect the input and output xmls mentioned above.
Sender Data Type and Message Type:
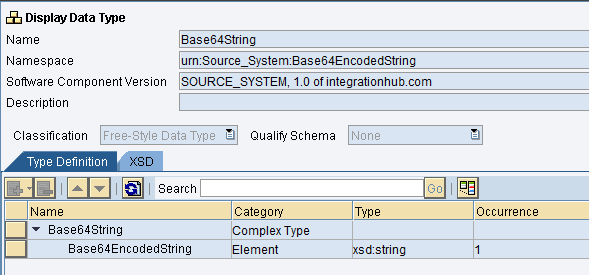
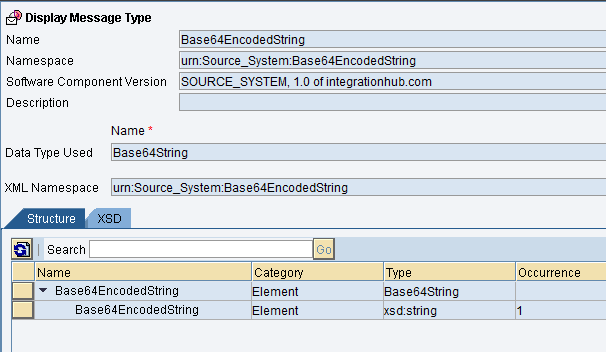
Receiver Data Type and Message Type:
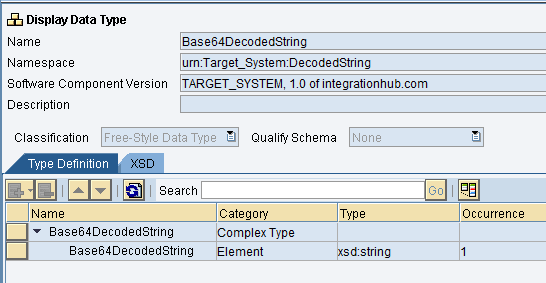
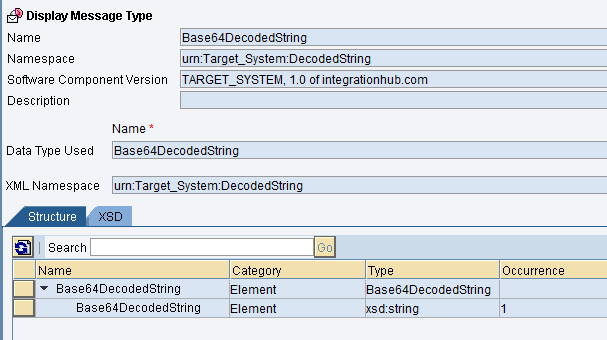
In this example we’ll use one outbound service interface for sender and one inbound service interface for receiver.
Outbound Service Interface:
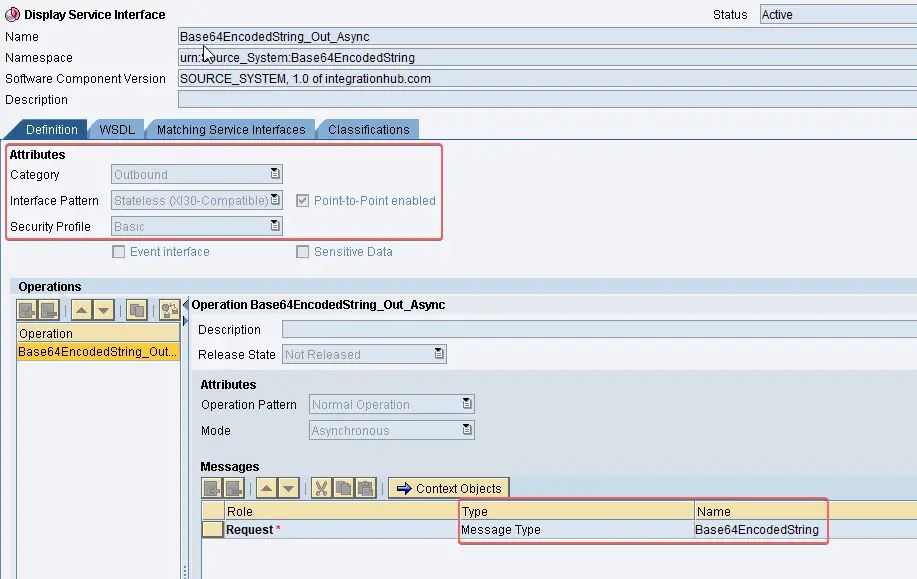
Inbound Service Interface:
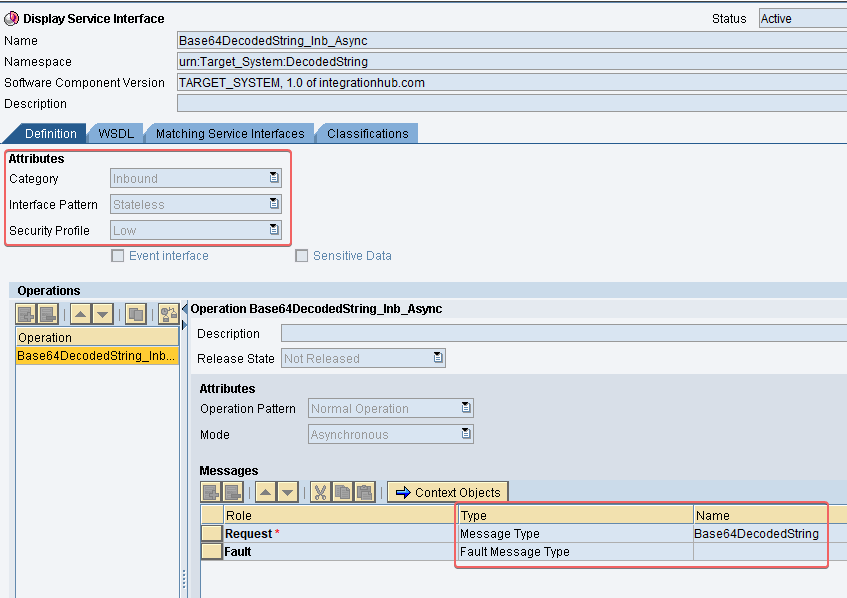
Step 2: Create Base64 Decoding Java Mapping in NWDS.
If you have not created Java Mappings in NWDS before, read my article on how to implement Java Mappings.
Implement the code snippet and export it as a .jar. Then import the .jar file as an Imported Archive to ESR.
package base64Decode; import java.io.InputStream; import java.io.OutputStream; import com.sap.aii.mapping.api.AbstractTransformation; import com.sap.aii.mapping.api.StreamTransformationException; import com.sap.aii.mapping.api.TransformationInput; import com.sap.aii.mapping.api.TransformationOutput; import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import org.w3c.dom.Document; import org.w3c.dom.Node; import java.util.Base64; public class Decoder extends AbstractTransformation { @Override public void transform(TransformationInput in, TransformationOutput out) throws StreamTransformationException { String encodedString = null; Node encodedStringNode; try { //Read input and output payloads. InputStream inputStream = in.getInputPayload().getInputStream(); OutputStream outputStream = out.getOutputPayload().getOutputStream(); //Creates new instance of DOM tree builder factory DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); //Creates new instance to obtain DOM document from xml. DocumentBuilder builder = factory.newDocumentBuilder(); //Obtain new DOM document from inputStream xml. Document doc = builder.parse(inputStream); //Obtain the XML node 'Base64EncodedString' of source message. encodedStringNode = doc.getElementsByTagName("Base64EncodedString").item(0); //Get the base64 string value of the xml element 'Base64EncodedString' from source message. encodedString = encodedStringNode.getFirstChild().getNodeValue(); //Decode base64 string to text. byte[] decodedBytes = Base64.getDecoder().decode(encodedString); String decodedString = new String(decodedBytes); //Derive output(target) xml message. outputStream.write(("<?xml version=\"1.0\" encoding=\"UTF-8\"?><ns1:Base64DecodedString xmlns:ns1=\"urn:Target_System:DecodedString\"><Base64DecodedString>"+decodedString+"</Base64DecodedString></ns1:Base64DecodedString>").getBytes()); } catch (Exception exception) { getTrace().addDebugMessage(exception.getMessage()); throw new StreamTransformationException(exception.toString()); } } }
Step 3: Create Imported Archive and Operation Mapping (OM).
Create Imported Archive by uploading Java Mapping class defined in step 2 as a .jar to ESR. Then configure the Operation Mapping with the Imported Archive.
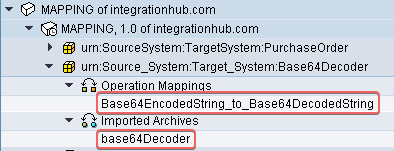
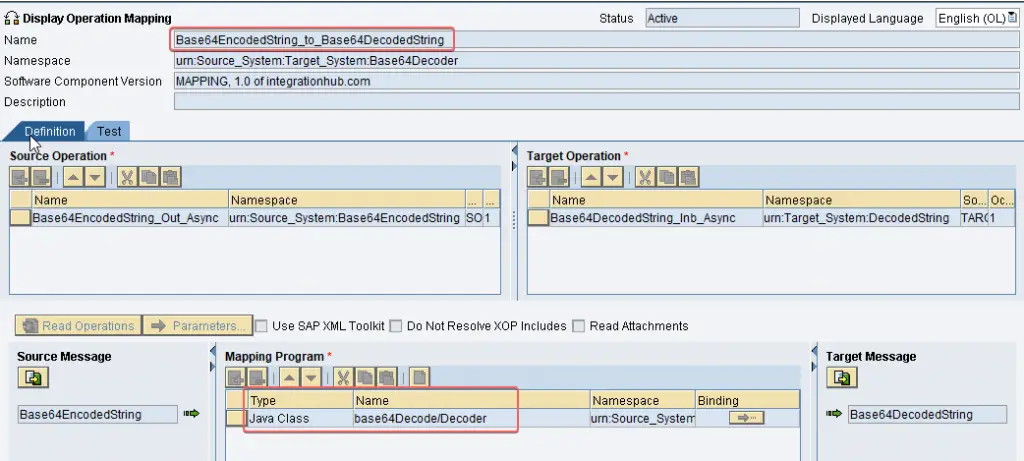
Test Base64 Decoding Java Mapping:
You can test Java Mapping using Operation Mapping test tool in ESR.
Base64 encoded string is ‘VGVzdGluZyBkZWNvZGVyLg==’.
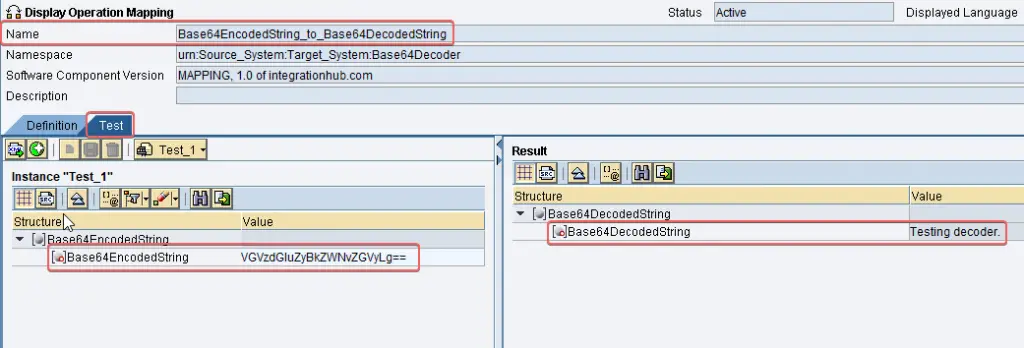
How to Encode Message to Base64 in SAP Integration Suite (CPI)
Hopefully, you will be able to reuse the structure of this Base64 decoding Java Mapping class for integration scenarios. Please, leave a comment below if you have any questions about Base64 decoding or Java Mappings.
can i use this on sap pi 7.4?