This article provides a Java mapping which encodes Base64 in SAP PI/PO. I will implement a simple interface to demonstrate the use of the Java mapping. It’s part of a series of posts about Base64 encoding and decoding and you can check the Base64 decoding Java Mapping example in my previous article.
Similarly, you can also use User Defined Functions (UDF) to encode and decode Base64 in SAP PI/PO. But in this article, I’ll show you how to use Java mapping for encoding.
SAP Versions used in the illustration:
- SAP PO 7.5
Base64 Encoding Java Mapping Scenario:
Source message node ‘TextMessage’ contains a string value that should be encoded. Base64 encoded string should be mapped to target message node ‘Base64EncodedString’.
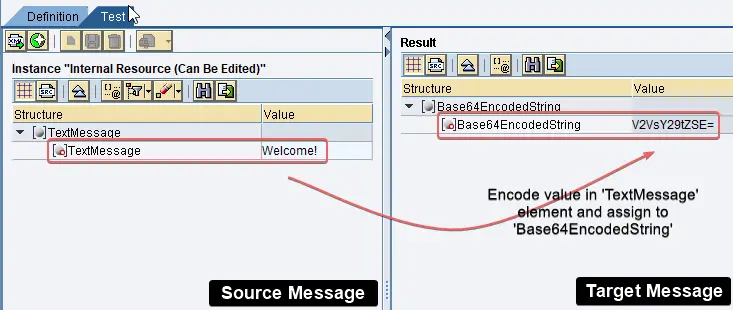
String ‘Welcome!’ translates to ‘V2VsY29tZSE=’ in Base64 schema.
System Detail Example was Implemented:
This example will be implemented in an SAP Process Integration (PI) system version 7.50 single stack.
Functionality of Java Mapping:
First functionality of Java mapping is to encode the text in Base64 schema. String value in sender message node ‘TextMessage’ should be encoded and included in target message element ‘Base64EncodedString’.
Then, Java mapping should create a complete target message xml with other elements, namespaces, etc.
If the target system is an SAP system, you can decode a Base64 string using ABAP Function Modules (FM) or ABAP class CL_HTTP_UTILITY. You can read more about how to encode or decode Base64 in SAP in my previous post.
Input Message:
<?xml version="1.0" encoding="UTF-8"?> <ns0:TextMessage xmlns:ns0="urn:Source_System:TextMessage"> <TextMessage>Welcome!</TextMessage> </ns0:TextMessage>
Output Message:
<?xml version="1.0" encoding="UTF-8"?> <ns1:Base64EncodedString xmlns:ns1="urn:Target_System:Base64EncodedString"> <Base64EncodedString>V2VsY29tZSE=</Base64EncodedString> </ns1:Base64EncodedString>
How to Implement Java Mapping in Enterprise Resource Repository (ESR):
Follow these steps to implement the Base64 encoding Java mapping.
- Create Data Types, Message Types and Inbound/Outbound Interfaces.
- Implement Base64 Encoder Java Class in Netweaver Developer Studio (NWDS).
- Define the Imported Archives and Operations Mapping (OM) in ESR.
Step 1: Create Data Types, Message Types and Inbound/Outbound Service Interfaces:
Create sender message and target message Data types and Message Types. Then, create inbound and outbound service interfaces.
Sender Message, Data Type and Message Type:
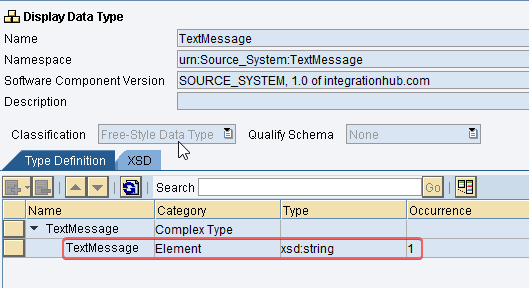
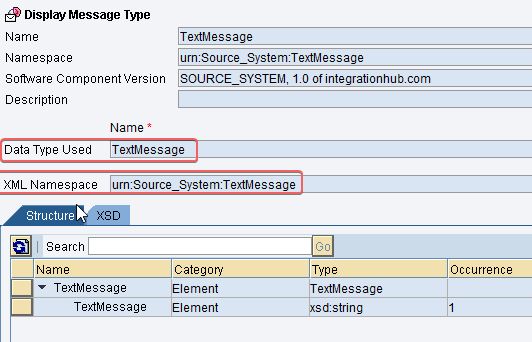
Target Message, Data Type and Message Type:
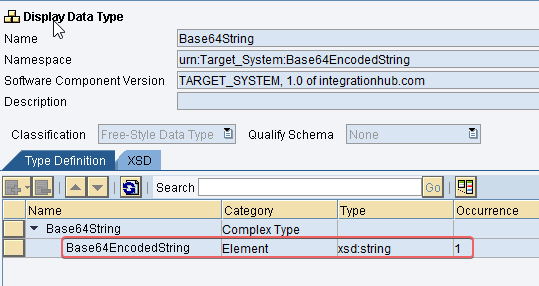
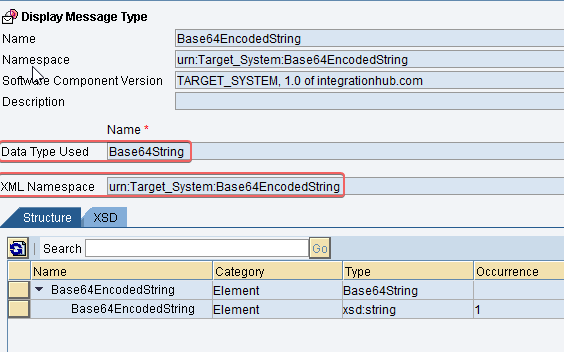
Inbound Service Interface:
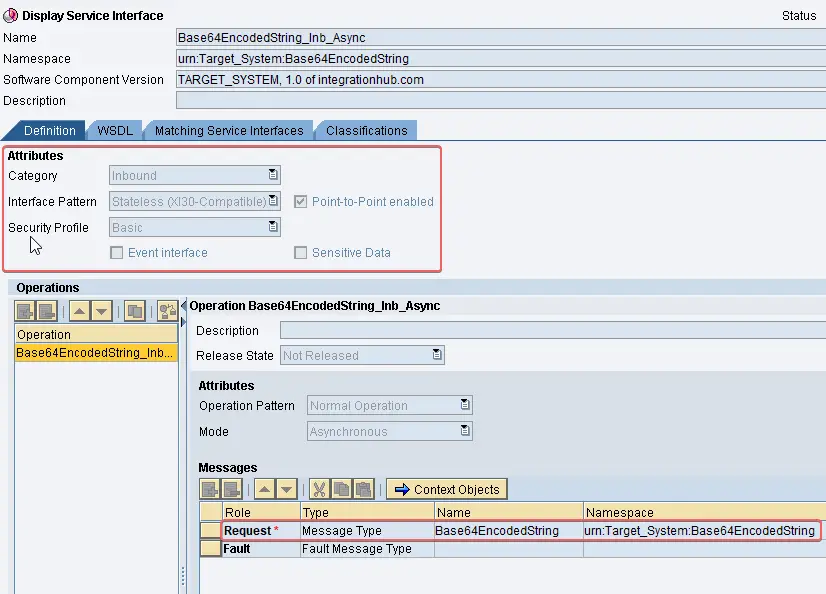
Outbound Service Interface:
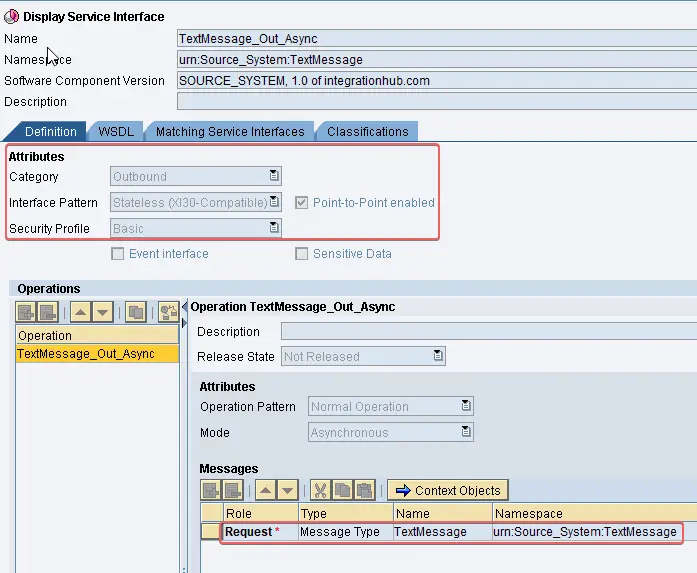
Step 2: Implement Base64 Encoder Java Class in NWDS:
We will use java.util.Base64 class to implement this mapping program.
Create the Encoder Java mapping class in NWDS. Export the implemented Java class as a .jar file.
package Base64Encoder; import java.io.InputStream; import java.io.OutputStream; import com.sap.aii.mapping.api.AbstractTransformation; import com.sap.aii.mapping.api.StreamTransformationException; import com.sap.aii.mapping.api.TransformationInput; import com.sap.aii.mapping.api.TransformationOutput; import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import org.w3c.dom.Document; import org.w3c.dom.Node; import java.util.Base64; public class Encoder extends AbstractTransformation { @Override public void transform(TransformationInput in, TransformationOutput out) throws StreamTransformationException { String textMessage = null; String encodedString = null; Node textMessageNode; try { //Read input and output payloads. InputStream inputStream = in.getInputPayload().getInputStream(); OutputStream outputStream = out.getOutputPayload().getOutputStream(); //Creates new instance of DOM tree builder factory DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); //Creates new instance to obtain DOM document from xml. DocumentBuilder builder = factory.newDocumentBuilder(); //Obtain new DOM document from inputStream xml. Document doc = builder.parse(inputStream); //Obtain the XML node 'TextMessage' of source message. textMessageNode = doc.getElementsByTagName("TextMessage").item(0); //Get the value of the xml element 'TextMessage' from source message. textMessage = textMessageNode.getFirstChild().getNodeValue(); //Encode text to base64 string. byte[] bytes = textMessage.getBytes(); encodedString = Base64.getEncoder().encodeToString(bytes); //Derive output(target) xml message. outputStream.write(("<?xml version=\"1.0\" encoding=\"UTF-8\"?><ns1:Base64EncodedString xmlns:ns1=\"urn:Target_System:Base64EncodedString\"><Base64EncodedString>"+encodedString+"</Base64EncodedString></ns1:Base64EncodedString>").getBytes()); } catch (Exception exception) { getTrace().addDebugMessage(exception.getMessage()); throw new StreamTransformationException(exception.toString()); } } }
Step 3: Create Imported Archive and Operation Mapping (OM):
Upload Java mapping as an Imported Archive (.jar) to ESR. Next, create Operation Mapping including the Imported Archive.
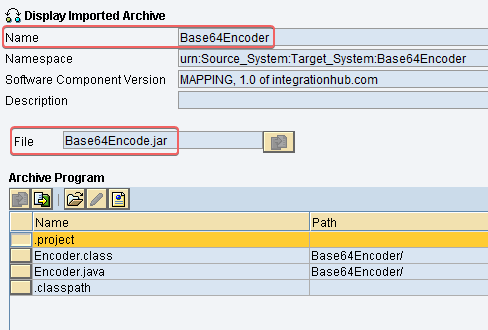
Configure Operation Mapping with Java class.
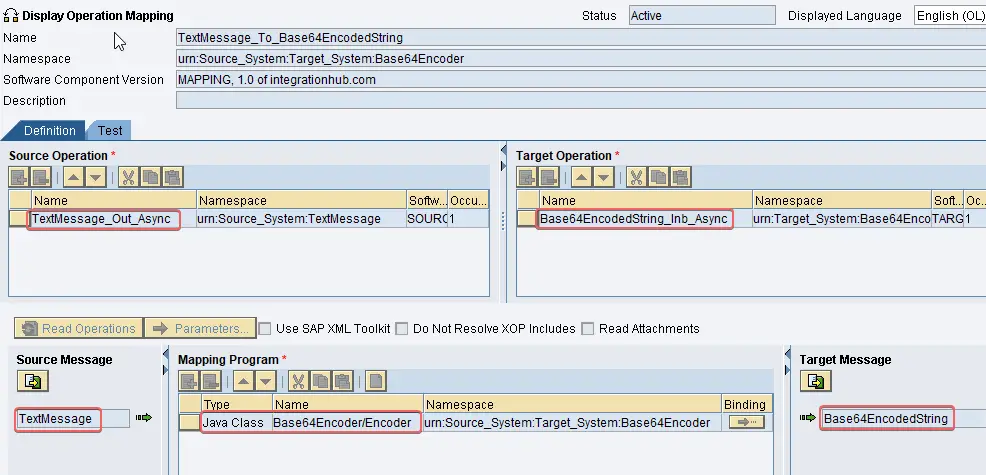
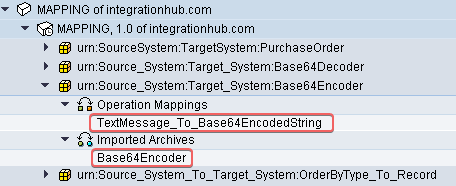
Test Base64 Encoder Java Mapping:
Test the Java Mapping program via Operation Mapping test tool.
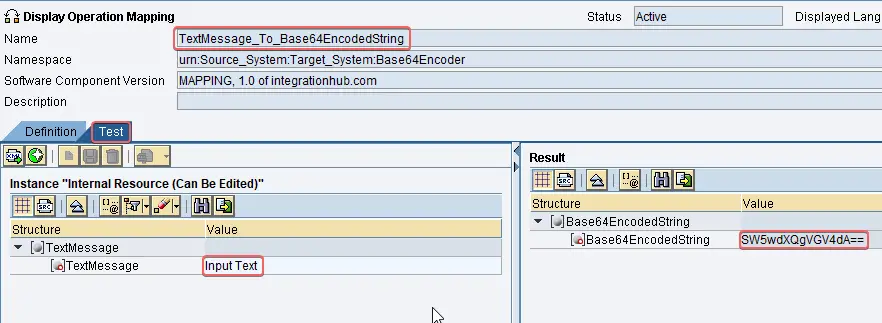
You can reuse Base64 encoding Java class with minor adjustments to suite your scenario. If you have any questions, please leave a comment below.
HI Isuru Fernando
I am trying to use this java mapping to pick the PDF file and convert to Base64.
Can you help me .
//Read input and output payloads.
InputStream inputStream = in.getInputPayload().getInputStream();
OutputStream outputStream = out.getOutputPayload().getOutputStream();
//Creates new instance of DOM tree builder factory
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
//Creates new instance to obtain DOM document from xml.
DocumentBuilder builder = factory.newDocumentBuilder();
//Obtain new DOM document from inputStream xml.
Document doc = builder.parse(inputStream);
//Obtain the XML node ‘TextMessage’ of source message.
textMessage = doc.getElementsByTagName(“*”);
//Get the value of the xml element ‘TextMessage’ from source message.
//textMessage = textMessageNode.getFirstChild().getNodeValue();
//Encode text to base64 string.
byte[] bytes = new byte[inputStream.available()];
encodedString = Base64.getEncoder().encodeToString(bytes);